Some friendly advice to make your programming and your scripts/programs more efficient ...
- Keep all scripts/programs under your home to get them backed up automatically!
- When coding make backup copies or use GIT (installed on all of our servers).
- Give names which have a “meaning”. Also for email “subjects”!
- Master the editor you are using. Know how to search (forwards/backwards, case sensitive/insensitive, whole words, ...)
- Know how to find things ('find', 'grep')
- Write comments (so others have it easier to understand your code as well as your)
- Use indentation (mandatory in Python, also helps to read the code and find errors)
- Use colors (helps to read the code and find errors)
- Give names which have a “meaning” (for scripts/programs/variables). This also applies for email “subjects”!

- Verify(!) what you are doing
When scripting, try out every line in command line (copy – paste), put prints/echo
When programming in i.e. Fortran put prints
Check intermediate files / results - Break big tasks up into smaller tasks - if possible. For example, if you want to plot daily means from hourly data, write one script to create the daily data. Verify that the new files are correct. Then write a Matlab/Python script that only plots the data.
- Use available tools: r.diag/editfst (for RPN files) and CDO/NCO (for NetCDF files)
- Error messages are not always at the end.
- Reduce memory usage:
- Matlab/Python: use 32-bit instead of 64-bit real/float numbers
- Do not load all data at the same time but only one file or even just one record at the time, treat it and then read the next record/file
- Reuse variables (this is more important than giving them meaningful names!) and/or
- Delete large arrays once you finished using them
- Monitor the cpu & memory usage of your jobs with 'top'. - Make use of the cache:
Accessing data in the cache is up to 100 times faster than accessing data in memory
=> Once you read data, use them before reading more data
Once you created a variable which you have to use again do this as soon as possible (before it gets moved out of the cache)
When using nested loops make sure to use them in the right order.
In languages which do not need to get compiled, like Matlab and Python, loops over elements of large arrays should be avoided as they are very time consuming.
If you have to loop over all the elements of an array make sure you nest the loops in the right order. Let's say you want to loop over all the elements of the array humi(ni,nj,nk). Then the order of your loops should be:
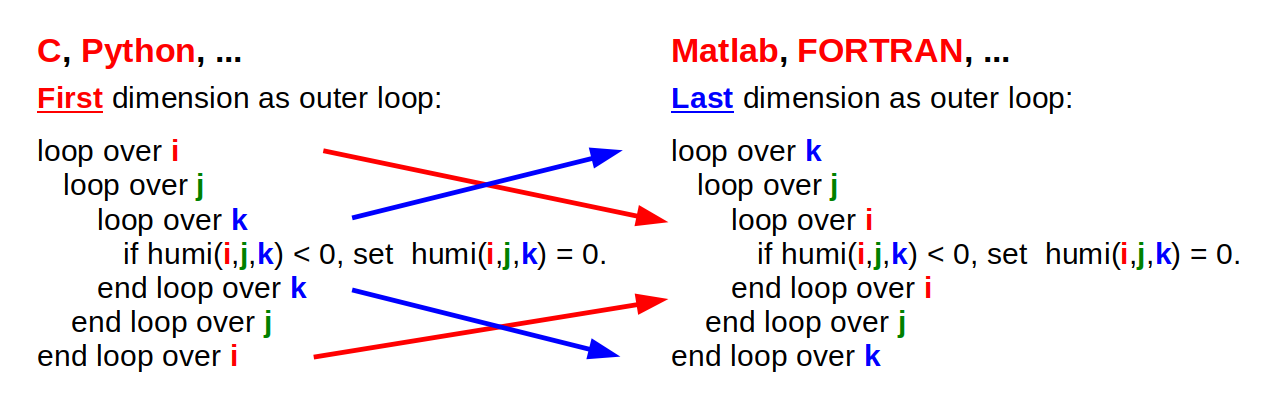